Discover the basic structure of Java program with our comprehensive guide. Learn the essential elements and create efficient code.
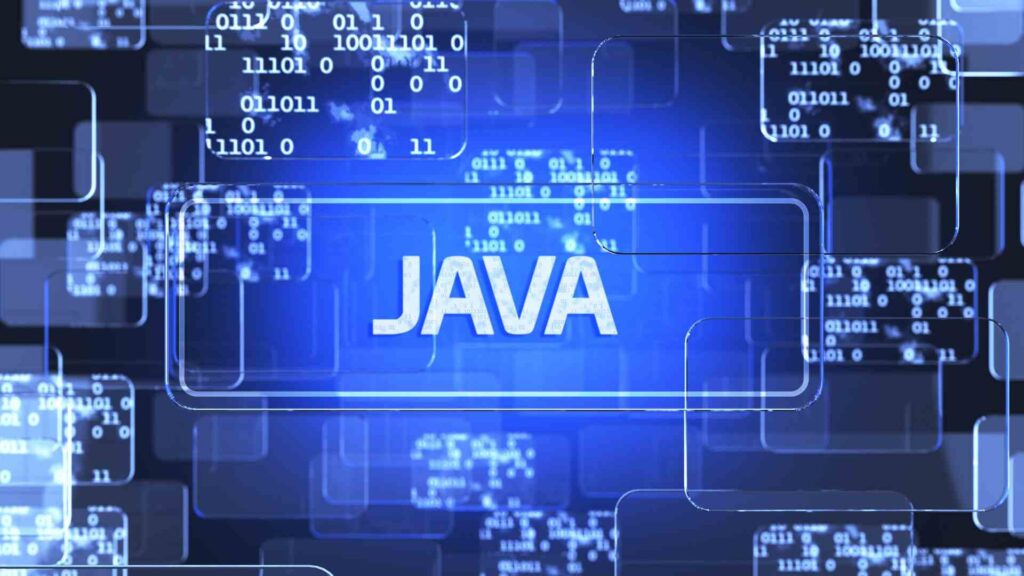
Basic Structure of Java Program Overview
Class Declaration
A Java program starts with a class declaration, defining the basic structure of java program and its behavior. This is where the main functionality is encapsulated.
Methods and Interface Declaration
Within the class, methods are defined to perform specific tasks. interface declaration allows for implementing multiple inheritances in Java programs.
Syntax and Entry Point
The syntax of a Java program follows specific rules to ensure proper execution. The entry point, known as the main
method, is where the program begins its execution.
Example Illustration
java public class HelloWorld { public static void main(String[] args) { System.out.println(“Hello, World!”); } }
In this simple example, a Java program prints “Hello, World!” to the console when executed.
Importance of Documentation Section
Including a documentation section in a Java program is crucial for providing clarity on the program’s purpose, functionality, and usage. It aids in understanding and maintaining the codebase effectively.
Documenting Your Java Program
Overview
When documenting your Java program, it is crucial to include descriptive comments within your code. These comments explain the purpose of each class, method, and section of code, aiding in understanding and maintenance.
Documentation Section
At the beginning of your source file, include a documentation section. This section serves as an overview of the Java program, detailing its functionalities and providing usage instructions for other developers.
JavaDoc Comments
Utilize JavaDoc comments in your code to automatically generate documentation. This feature simplifies the process for other developers to comprehend and utilize your Java program effectively.
- Pros:
- Facilitates better understanding for developers.
- Enhances code maintainability and collaboration.
- Cons:
- Requires additional time and effort during initial development.
- May become outdated if not regularly updated.
Including examples of input, output, and expected results in your documentation is essential. These examples help users grasp how to interact with your Java program efficiently.
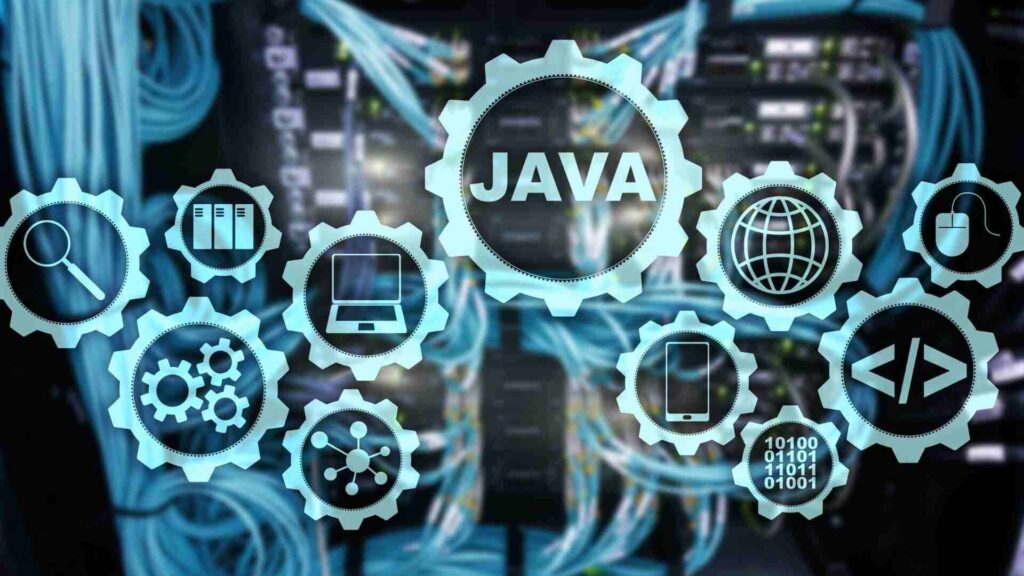
Defining Packages in Java
Syntax for Packages
When defining packages in Java, developers use the “package” keyword followed by the specific package name. This syntax helps organize classes effectively.
Importance of Packages
Packages in Java play a crucial role in avoiding naming conflicts and enhancing access protection within applications. They also contribute to code reusability.
Role in Java Programs
Packages are essential for grouping related classes and interfaces together. This grouping facilitates easier navigation and understanding of specific functionalities within a program.
Including External Code Snippets
Formatting Comments
When incorporating external code snippets in your Java program, it’s crucial to use comments effectively. These comments provide a concise description of each element, enhancing the readability of your code. By including comments within your code snippets, you can explain the purpose and functionality of different sections.
Organizing Functionalities
To showcase a clear structure in your Java program, organize the code snippets based on various functionalities or methods. This organization helps in presenting a systematic flow of the program’s logic and operations. By categorizing code snippets according to their specific functions, you can create a more coherent and understandable program structure.
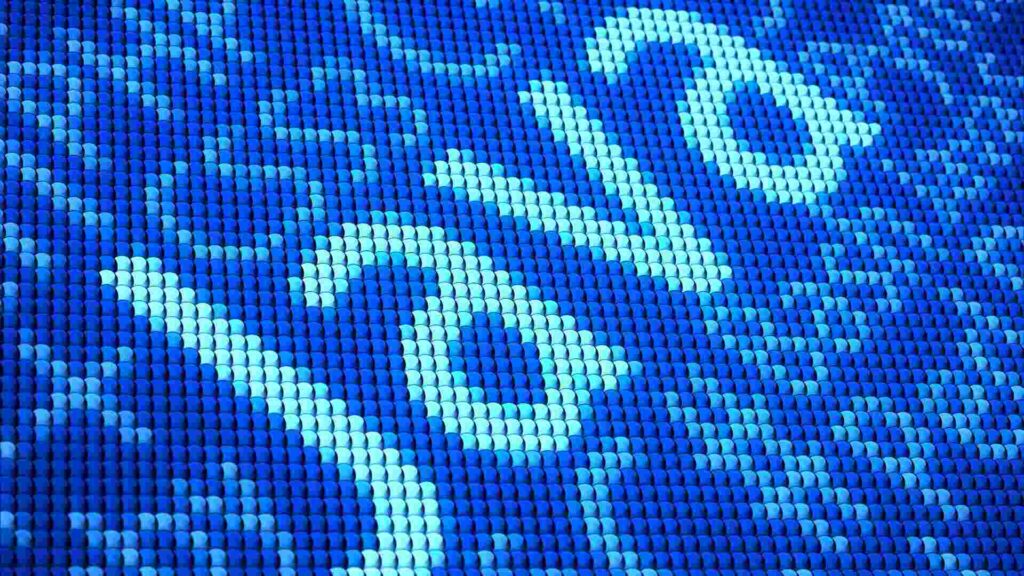
Creating Interfaces in Java
Interface Declaration
An interface in a Java program defines a set of methods that a class must implement. It serves as a contract for classes to follow, specifying the methods they need to provide.
Syntax and Creation
To declare an interface in Java, use the keyword “interface” followed by the interface name. For example: java public interface ExampleInterface { // method signatures }
Interfaces are created to enforce certain methods that classes implementing the interface must define.
Defining Methods for Implementation
Interfaces are crucial in Java as they allow for abstraction and standardization. By defining methods within an interface, any class implementing that interface must provide concrete implementations for those methods.
- Pros:
- Ensures consistency in method implementation across different classes.
- Allows for multiple inheritance-like behavior through interfaces.
- Cons:
- Classes can only extend one superclass but implement multiple interfaces.
Example Usage
Consider an interface called Shape
with a method calculateArea()
. Any class implementing this interface, such as Circle
or Rectangle
, must define how to calculate the area specific to that shape.
Defining Classes in Java
Class Declaration
To define a class in Java, use the “class” keyword followed by the specific class name like “class Student”. This declaration encompasses the class’s attributes, methods, and constructors to dictate the behavior of objects created from this class.
Class Functionality
Classes in Java act as blueprints for creating objects, consolidating data and methods within a single entity. This structuring ensures that each object instantiated from a class adheres to the defined structure and behavior.
Implementing Interfaces
When defining classes in Java, it is beneficial to incorporate interfaces to specify the expected functionality that the class should offer. By integrating interfaces, you enhance code modularity and reusability, facilitating a more organized and scalable codebase.
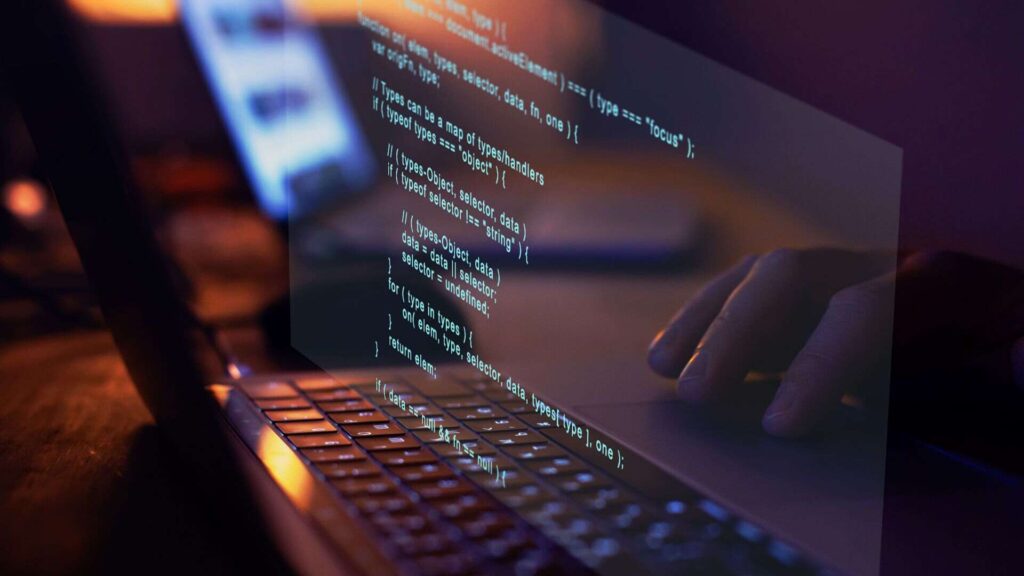
The Main Method Class
Class Declaration
In Java programming, the specific class known as the Main Method Class plays a crucial role. This class serves as the entry point for executing Java applications. It is essential to understand the significance of class declaration in defining the basic structure of a Java program.
When creating a Java program, developers must define classes to encapsulate data and functionality. Within these classes, methods are implemented to perform specific tasks. The class declaration specifies the properties and behaviors that objects of that class will exhibit.
Main() Method
The Main Method Class contains the main() method, which acts as the starting point for executing Java applications. This method is where the program begins its execution. By including the main() method within the Main Method Class, developers designate where the program should start running.
Developers must ensure that the class definition includes the main() method with the correct syntax and parameters to execute successfully. Understanding how to create and utilize the Main Method Class is fundamental in ensuring the proper functionality of a Java program.
Managing Variables and Constants
Declaring Variables
To store data within a Java program, programmers declare variables with specific data types like int, double, or String. These variables hold values that can be changed during the program’s execution.
Defining Constants
Constants are used for values that should remain unchanged throughout the program. By declaring constants, developers ensure that critical values stay consistent and do not accidentally get altered.
Passing Parameters
When passing parameters to methods in Java, developers enable dynamic changes and enhanced functionality within their programs. This allows for flexibility in executing actions based on different inputs.
Role in Program Structure
Variables and constants play a pivotal role in shaping the basic structure of a Java program. They provide a means to store, manipulate, and retrieve data efficiently, contributing to the overall functionality of the application.
Explaining Comments in Java
Syntax Overview
In Java programming, comments play a crucial role in enhancing readability and providing essential details about the code. To add comments, use double forward slashes (//) for single-line comments and /* */ for multi-line comments. These comments are vital for documenting the code structure and logic.
Usage Examples
Within class declarations, comments can describe the purpose of the class or provide additional context. In interface declarations, comments clarify the role of interfaces in the program’s architecture. When used within methods, comments explain the function’s behavior and parameters, aiding developers in understanding complex algorithms.
Importance of Descriptive Comments
Including comments to describe changes made in the codebase helps team members track modifications effectively. Providing instructions for execution through comments ensures seamless program operation. Acknowledging and crediting the author within the documentation section is a common practice that fosters collaboration and recognition within development teams.
Utilizing Braces in Programming
Organizing Code
Braces play a crucial role in Java programming by organizing code blocks within methods, classes, and interfaces. They define the structure of the program, ensuring clarity and readability.
When writing a Java program, braces are used to mark the beginning and end of code blocks. This delineation is essential for the proper execution flow within the program, guiding how different elements interact.
Correct Placement
In Java, braces are correctly placed when defining a class, implementing methods, or creating loop structures. For example, class declarations enclose their content within braces to define the class body accurately.
In method implementations, braces encapsulate the method’s functionality. Similarly, loop structures like “for” or “while” loops use braces to contain the statements that need to be executed repeatedly.
Importance of Consistency
Consistent usage of braces is critical in Java programming for enhancing readability, maintainability, and adherence to coding standards. By following a uniform style throughout the codebase, developers can easily navigate and understand the logic behind each block of code.
- Pros:
- Enhances code organization
- Facilitates debugging process
- Cons:
- Inconsistent brace placement can lead to syntax errors
Implementing Main Method Functionality
Main Method
The main method serves as the entry point for a Java program, starting the execution of the code. It is where the program begins its actions and proceeds with its functionalities.
Utilization of Methods
Methods in Java programs are crucial for performing specific actions. They enhance code readability by breaking down complex tasks into smaller, manageable parts. By utilizing methods, programmers can streamline their code and make it more organized.
Importance of Documentation
Proper documentation within the main method is essential for understanding the program’s functionality. Descriptive comments help other developers comprehend the purpose of each section, making it easier to maintain and modify the code in the future.
Creation and Execution of Java Applications
In Java, applications are created by writing code that specifies various actions to be performed. Once compiled and executed, these applications produce an output on the screen, showcasing the end result of the program’s operations.